You don’t need any pull up or pull down resistors, useĀ
pull_up_down=GPIO.PUD_DOWN
instead.
The setup:
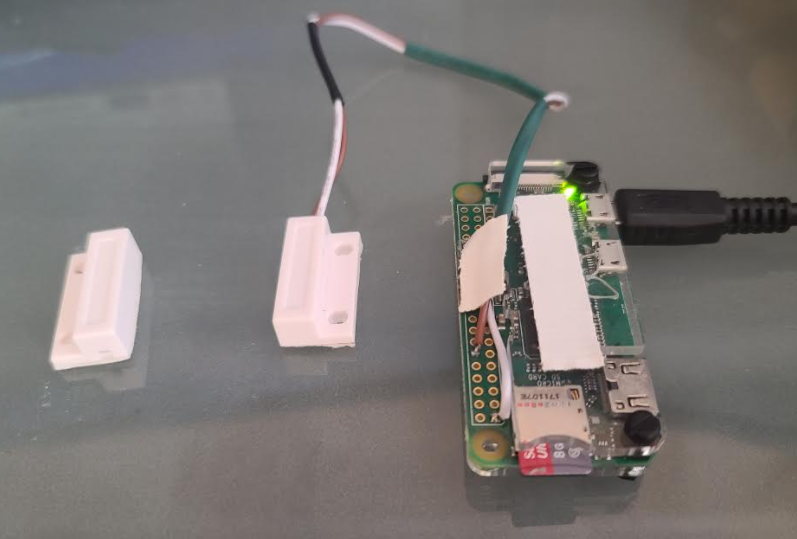
The wiring:
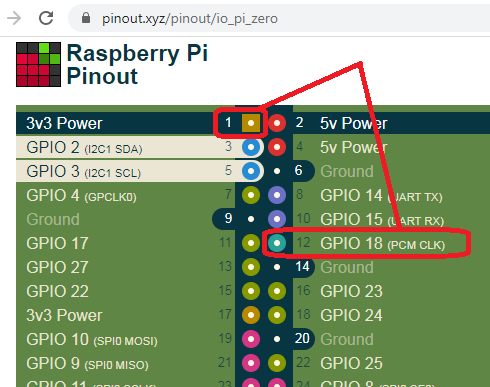
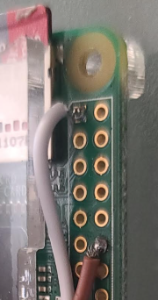
The test program: test.py
# Door open/closed detector # # (c) Alan Lupsha 2020 # wiki.lupsha.com import RPi.GPIO as GPIO import time GPIO.setmode(GPIO.BCM) # GPIO Numbers instead of board numbers MAGNET_GPIO = 18 GPIO.setup(MAGNET_GPIO, GPIO.IN, pull_up_down=GPIO.PUD_DOWN) # GPIO Assign mode print("monitoring in progress...") try: while (True): print('MAGNET_GPIO is: %s' % GPIO.input(MAGNET_GPIO)) time.sleep(0.1) # If user presses ^C cleanup the GPIO except KeyboardInterrupt: GPIO.cleanup() print("Exiting program, bye.")
Run it.
python3 test.py
Open:
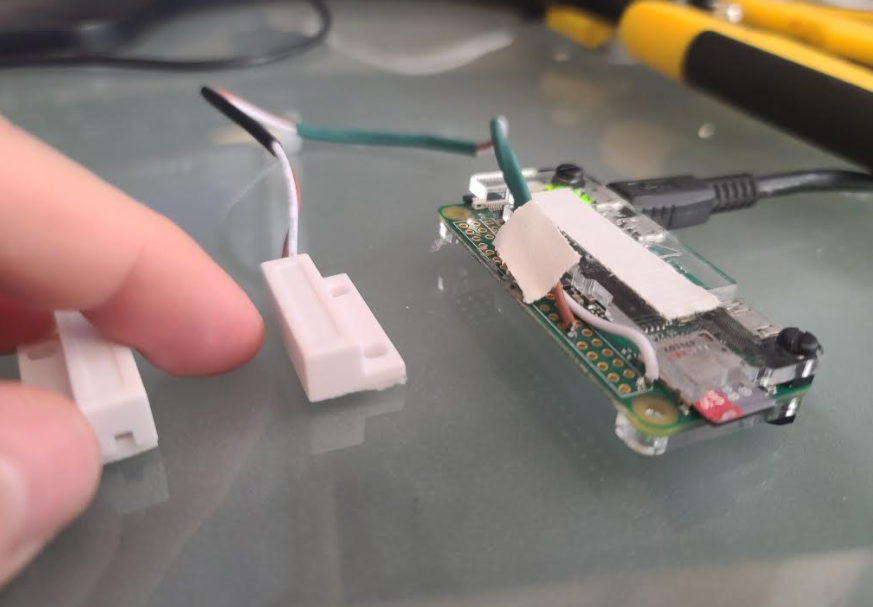
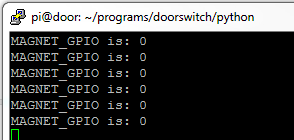
Closed:
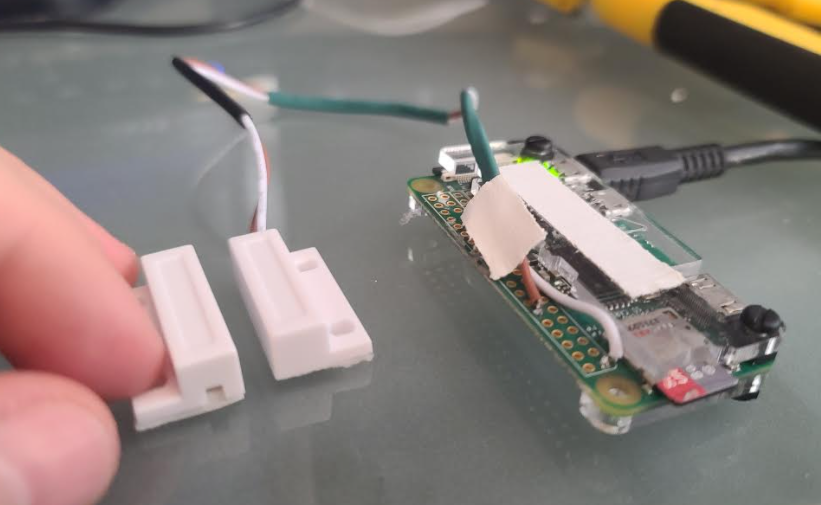
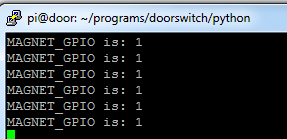
A more complex example: (2 files)
door.py
# Door open/closed detector # # This programs monitors a reed switch connected to GPIO 18 and 3VDC # When the reed switch is open, the sendalert method is called, which # contacts other alarm systems. When the reed switch is closed, the # alarm mode is reset and sendstopalert is called. # # (c) Alan Lupsha 2020 # wiki.lupsha.com import RPi.GPIO as GPIO import time from datetime import datetime import urllib3 import requests, json #import http.client as httplib #import sys import ledstuff GPIO.setmode(GPIO.BCM) # GPIO Numbers instead of board numbers MAGNET_GPIO = 18 GPIO.setup(MAGNET_GPIO, GPIO.IN, pull_up_down=GPIO.PUD_DOWN) # GPIO Assign mode #LED_GPIO = 23 #GPIO.setup(LED_GPIO, GPIO.OUT) # the red LED # initially there is no alert alert = False def loginfo(message): rightnow = datetime.now().strftime("[%Y/%m/%d %H:%M:%S]") print(rightnow, ' [INFO] ', message) return def logerror(message): rightnow = datetime.now().strftime("[%Y/%m/%d %H:%M:%S]") print(rightnow, ' [ERROR] ', message) return def logexception(message, exception): rightnow = datetime.now().strftime("[%Y/%m/%d %H:%M:%S]") print(rightnow, ' [ERROR] ', message, ' ==> ', exception) return def urlhit(httpurl, userMessage): sample = { "message": userMessage } jsonData = json.dumps( sample ) loginfo('urlhit %s with message %s' % ( httpurl, jsonData)) try: response = requests.post(httpurl, jsonData) except urllib3.exceptions.NewConnectionError as e: logexception('new connection error 1 yo', e) except urllib3.exceptions.MaxRetryError as e: logexception('max retry error 2 yo', e) except requests.exceptions.ConnectionError as e: logexception('connection error 3 yo', e) return def startalert( str ): # GPIO.output(LED_GPIO, True) # Send an alert into the cloud now = datetime.now() rightnow = now.strftime("[%Y/%m/%d %H:%M:%S]") message = "Door open at " + rightnow loginfo(message) urlhit('http://localhost:8080/door/open', message) return def stopalert( str ): # GPIO.output(LED_GPIO, False) # Send END of alert into the cloud now = datetime.now() rightnow = now.strftime("[%Y/%m/%d %H:%M:%S]") message = "Door closed at " + rightnow loginfo(message) urlhit('http://localhost:8080/door/close', message) return loginfo("monitoring in progress...") ledstuff.arm() totalcounts = 0 try: while (True): totalcounts = totalcounts + 1 # MAGNET_GPIO = 1 means the circuit is closed, there is NO alert, = 0 means ALERT # print('******************** MAGNET_GPIO is: %s' % GPIO.input(MAGNET_GPIO)) # a break took place just now if alert == False and GPIO.input(MAGNET_GPIO) == 0: alert = True startalert( time ) loginfo('=> alert now is: DOOR OPEN') ledstuff.ledon() # an ongoing breach is STILL taking place if alert == True and GPIO.input(MAGNET_GPIO) == 0: # loginfo('=> breach still taking place') ledstuff.ledon() # the breach has ended, we are resetting the alert mode as well if alert == True and GPIO.input(MAGNET_GPIO) == 1: alert = False # reset it stopalert( time ) loginfo('=> alert is reset, door is now CLOSED') # there is no breach, sensor is closed #if alert == False and GPIO.input(MAGNET_GPIO) == 1: # loginfo('=> all ok, GPIO.INPUT(MAGNET_GPIO) is %s' % GPIO.input(MAGNET_GPIO)) # every x times, blink the LED if totalcounts == 10: ledstuff.blink() totalcounts = 0 # wait a bit time.sleep(1) # If user presses ^C cleanup the GPIO except KeyboardInterrupt: GPIO.cleanup() loginfo("Exiting program, bye.")
ledstuff.py
# LED on/off tester # (c) Alan Lupsha 2020 # wiki.lupsha.com import RPi.GPIO as GPIO import time GPIO.setmode(GPIO.BCM) # GPIO Numbers instead of board numbers LED_GPIO = 21 GPIO.setup(LED_GPIO, GPIO.OUT) # GPIO Assign mode def ledon(): #print('led ON') GPIO.output(LED_GPIO, True) return def ledoff(): #print('led OFF') GPIO.output(LED_GPIO, False) return # short blinks every X seconds def blink(): ledon() time.sleep(0.1) ledoff() return def angry(): ledon() time.sleep(0.5) ledoff() return # blink a number of times per second, to signify alertness def blinkpersecond(timestoblink): delay = 1 / timestoblink for i in range( 0, timestoblink ): #print('delay = ' + str(delay) + ' blink = ' + str(i)) ledon() time.sleep(delay) ledoff() time.sleep(delay) return # arming def arm(): blinkpersecond(2) blinkpersecond(5) blinkpersecond(10) ledoff() return def disarm(): ledoff() return