The final product
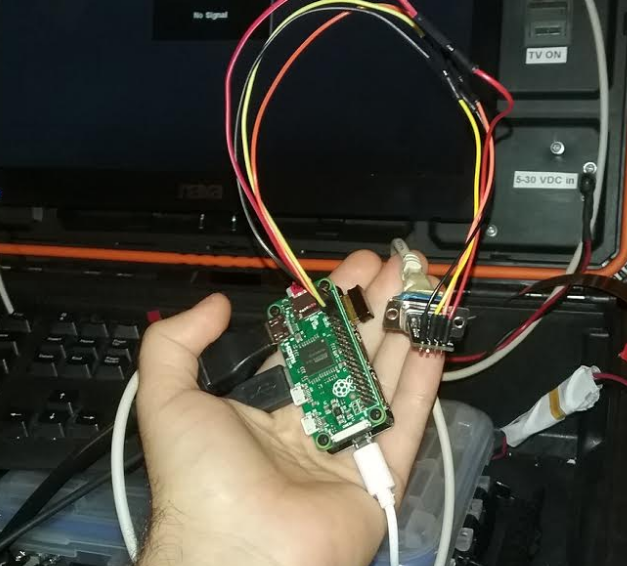
Contents
Setting up serial on the RPi
Enable serial communication and disable login over serial, which you likely don’t need.
sudo nano /boot/config.txt # add this line at the bottom, or make sure it's set to 1: enable_uart=1 # reboot shutdown -r now # remove the login service over serial sudo systemctl stop serial-getty@ttyAMA0.service sudo systemctl disable serial-getty@ttyAMA0.service # edit cmdline and remove the line that looks like this: console=serial0,115200 sudo nano /boot/cmdline.txt # example contents of cmdline.txt: cat /boot/cmdline.txt dwc_otg.lpm_enable=0 console=tty1 root=/dev/mmcblk0p2 rootfstype=ext4 elevator=deadline fsck.repair=yes rootwait ipv6.disable=1 # reboot shutdown -r now # check that /dev/serial points to ttyAMA0 ls -l /dev/serial* # output: lrwxrwxrwx 1 root root 7 Feb 1 22:15 /dev/serial0 -> ttyAMA0 Credits: http://spellfoundry.com/2016/05/29/configuring-gpio-serial-port-raspbian-jessie-including-pi-3/
My serial hardware – an 8 port relay box
In my case, I need to connect to this 8-relay box:
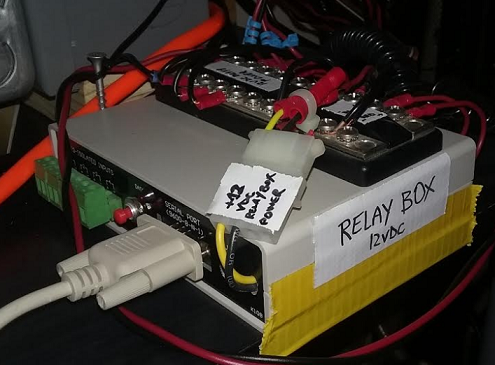
(purchased from: QuasarElectronics.co.uk )
and issue commands to turn on any of the 8 relays, using the on commands: (N1, N2, … N8), or off commands (F1, F2, … F8 )
Testing in Windows
In Windows this works right off the bat, with a regular serial cable, and this realterm program from https://sourceforge.net/projects/realterm/ :
Download a terminal application and change the baud rate to 9600, and OPEN the serial connection.
To turn ON relay 1, use N1, to turn it off, use N0. Example run from Windows to Quasar Electronics relay box, actually working:
Buy a converter
Like this male one: https://www.amazon.com/gp/product/B00OPU2QJ4/ref=oh_aui_detailpage_o03_s00?ie=UTF8&psc=1
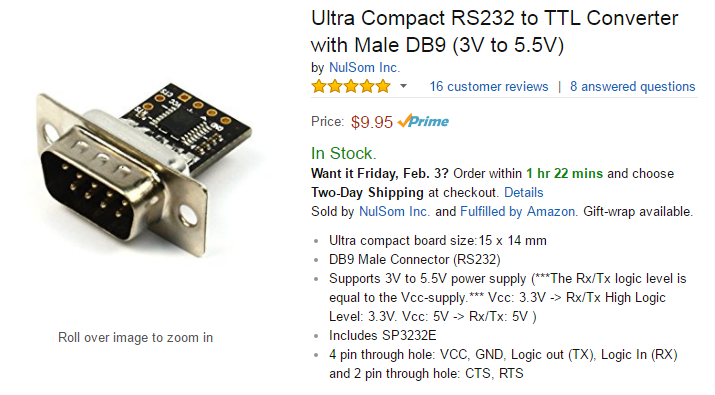
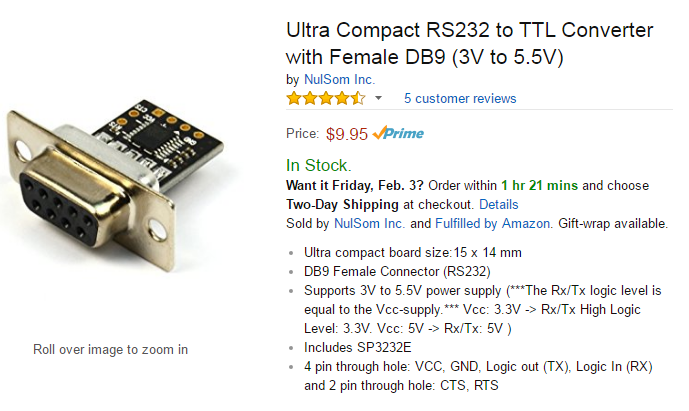
Solder some wires
Wire up the converter, VCC, ground, transmit, and receive.
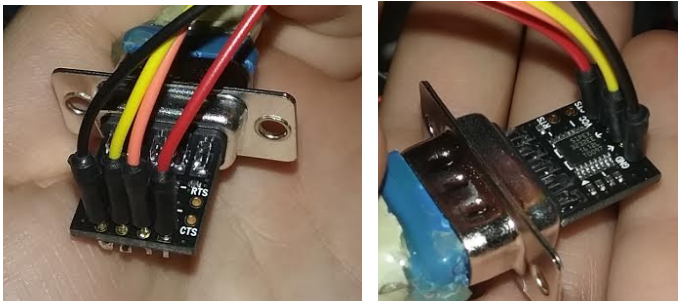
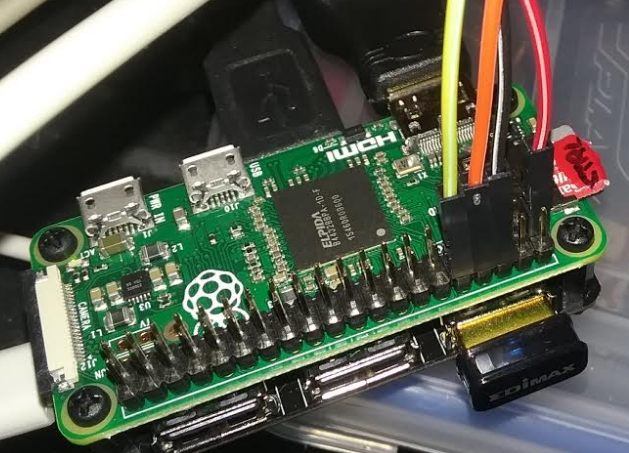
If you bought the male converter, you wire up the converter’s transmit to the RPi receive, and the converter’s receive (RX) to the RPi transmit (TX). If you bought the female converter, you wire them up one-to-one, transmit to transmit, receive to receive.
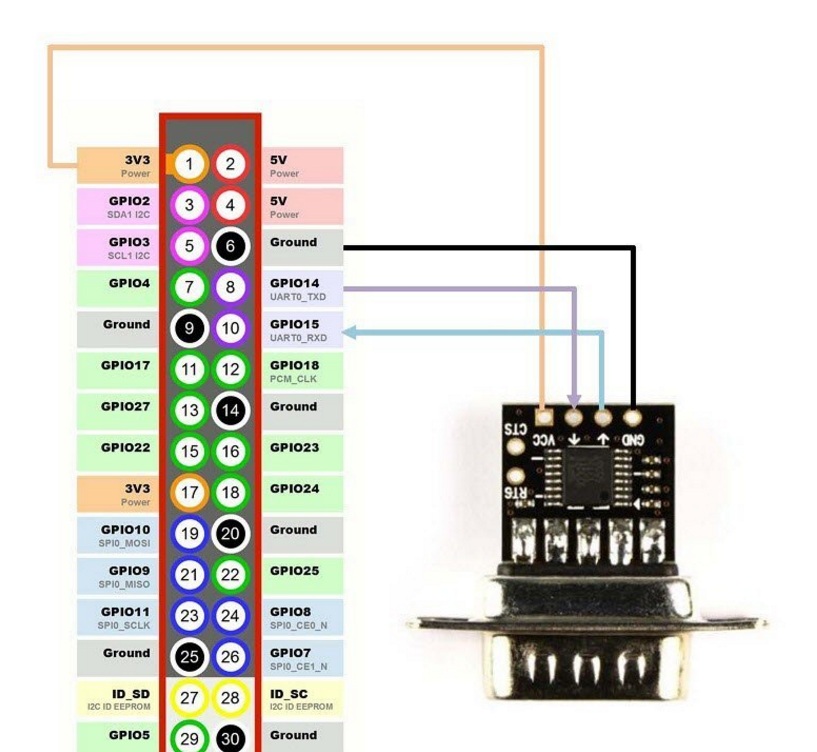
Issue serial commands from the RPi
Use the “screen” command:
screen /dev/serial0 9600
You won’t see what you’re typing, since echo is likely off. I tested passing the “turn on relay 1 command”, or “N1”, and then off, “F1”, as simple text, and it works.
To get out of the “screen” program, you type Control + A , D
Using minicom and then giving up
You can also install minicom: “apt-get install minicom” and then issue commands:
minicom --baudrate 9600 --device /dev/serial0 --8bit
which ignores your settings, and you have to go to options (Control A O )
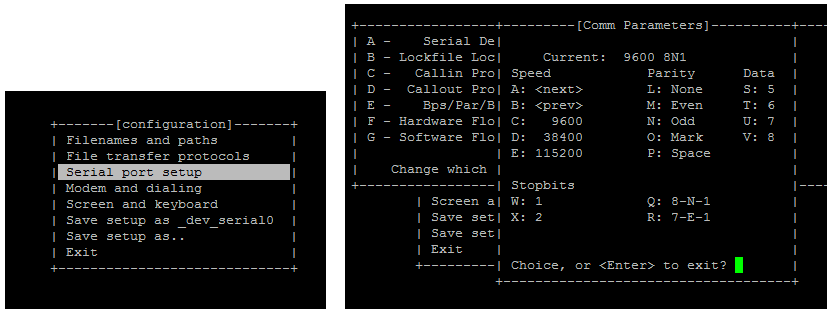
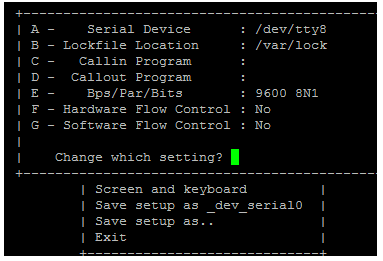
and don’t forget to type A and change /dev/tty8 to /dev/serial0 , of course you’ll forget.
And then you’ll still be offline, because this doesn’t work, and you decide that using “screen” is better, and you can automate that with scripts.
To exit minicom, Control + A, x, <enter>
Scripts to automate it all
This creates a screen session, and saves its name in a file. Runs only one time, at reboot. All other scripts to turn on/off relays use this screen session.
touch /home/pi/startscreen.sh chmod a+x /home/pi/startscreen.sh nano /home/pi/startscreen.sh
#!/bin/bash sudo chmod a+rw /dev/serial0 # store session in file myScreenSession="alan1" echo "$myScreenSession" > /home/pi/session.txt # set up session screen -dmS "alan1" /dev/serial0 9600 # list screens screen -list
Edit your crontab to set it up to run at reboot
crontab -e
and set its contents to:
@reboot /home/pi/startscreen.sh
Try it manually as well:
/home/pi/startscreen.sh
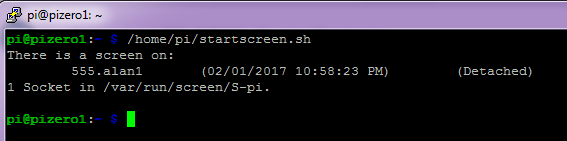
Reboot: sudo shutdown -r now
Check to see that the screen is running:
screen -list

Set up bash scripts to turn ON or OFF each relay
Try it manually to make sure it works. We’ll issue “screen” “session name” “command” and then the <ENTER> character. And we must not forget the semicolon at the end.
screen -S "alan1" -X stuff "N1"`echo -ne '\015'`;
… and relay 1 clicks on, and the beacon turns.
Let’s quickly turn it off:
screen -S "alan1" -X stuff "F1"`echo -ne '\015'`;
Relay 1 on, we’ll call this “on1.sh”, and relay 1 off, we’ll call this “off1.sh”
touch /home/pi/on1.sh chmod u+x /home/pi/on1.sh nano /home/pi/on1.sh
#!/bin/bash myScreenSession=`cat /home/pi/session.txt` if [[ -z "$myScreenSession" ]]; then echo "myScreenSession is not defined" else echo "Ok, myScreenSession is: $myScreenSession" screen -S "$myScreenSession" -X stuff "N1"`echo -ne '\015'`; fi
and the off script:
touch /home/pi/off1.sh chmod u+x /home/pi/off1.sh nano /home/pi/off1.sh
#!/bin/bash myScreenSession=`cat /home/pi/session.txt` if [[ -z "$myScreenSession" ]]; then echo "myScreenSession is not defined" else echo "Ok, myScreenSession is: $myScreenSession" screen -S "$myScreenSession" -X stuff "F1"`echo -ne '\015'`; fi
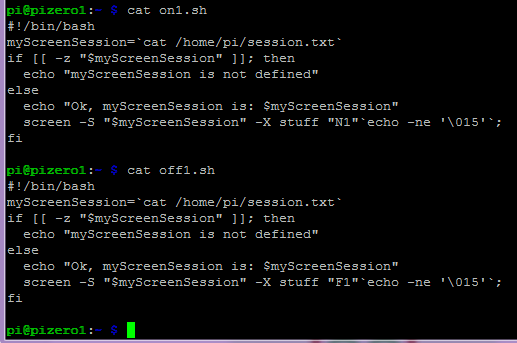
Execute them:
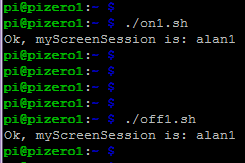