/var/www/cam$ ls -la
total 20
drwxr-xr-x 3 root root 4096 Dec 12 2014 .
drwxr-xr-x 3 root root 4096 Feb 24 00:00 ..
-rw-r--r-- 1 root root 5795 May 20 00:03 index.html
drwxr-xr-x 2 root root 4096 Dec 12 2014 js
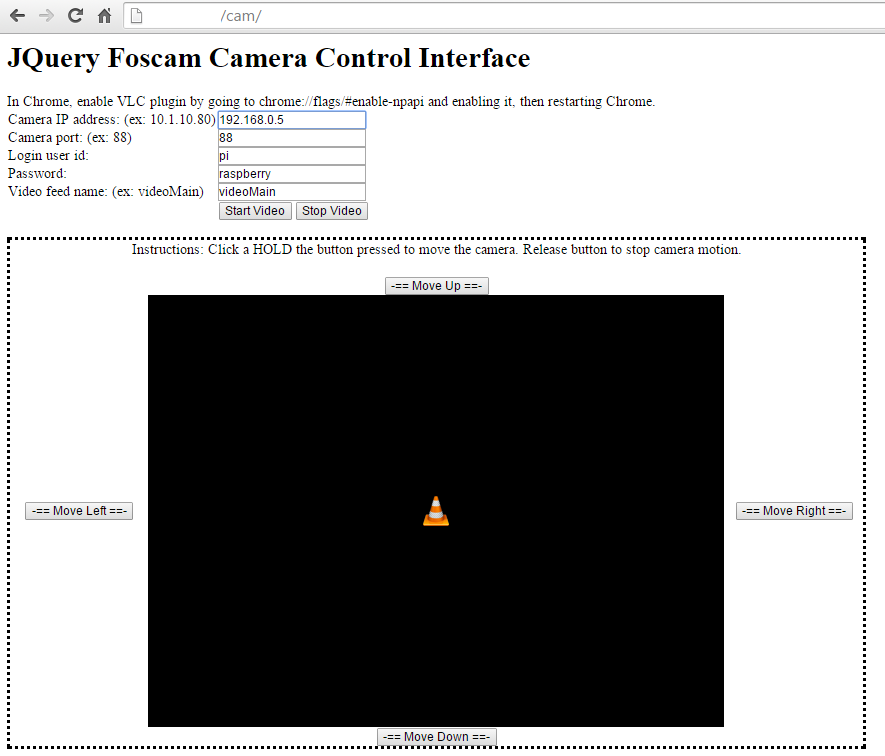
index.html:
<html>
<head>
<script src="./js/jquery-2.1.1.min.js"></script>
<style>
.wide {
width: 150px;
text-align: center;
}
.outlined {
border: dotted;
cellpadding: 10px;
}
</style>
</head>
<body>
<script type="text/javascript">
$(document).ready(function () {
$("#buttonLeft").mousedown(function () {
ajaxMove('left');
});
$("#buttonLeft").mouseup(function () {
ajaxMove('stop');
});
$("#buttonRight").mousedown(function () {
ajaxMove('right');
});
$("#buttonRight").mouseup(function () {
ajaxMove('stop');
});
$("#buttonUp").mousedown(function () {
ajaxMove('up');
});
$("#buttonUp").mouseup(function () {
ajaxMove('stop');
});
$("#buttonDown").mousedown(function () {
ajaxMove('down');
});
$("#buttonDown").mouseup(function () {
ajaxMove('stop');
});
$("#startVideoButton").click(function () {
$rtspUrl = 'rtsp://' + $("#login").val() + ':' + $("#password").val() + '@' +
$("#ipAddress").val() + ":" + $("#cameraPort").val() + '/'+ $("#videoFeedName").val();
console.log("rtspUrl is : " + $rtspUrl);
// getting parent for further use
embedParent = $("#vlc").parent();
// remove element from DOM and re-attach
embed = $("#vlc").detach();
embed.attr("target", $rtspUrl);
embed.appendTo(embedParent);
});
$("#stopVideoButton").click(function () {
// getting parent for further use
embedParent = $("#vlc").parent();
// remove element from DOM and re-attach
embed = $("#vlc").detach();
embed.attr("target", "");
embed.appendTo(embedParent);
});
});
ajaxMove = function ($direction) {
$url = 'http://' + $("#ipAddress").val() + ":" + $("#cameraPort").val() +
'/cgi-bin/CGIProxy.fcgi?cmd=';
if ($direction == 'left') {
$url += 'ptzMoveLeft';
}
else if ($direction == 'right') {
$url += 'ptzMoveRight';
}
else if ($direction == 'up') {
$url += 'ptzMoveUp';
}
else if ($direction == 'down') {
$url += 'ptzMoveDown';
}
else if ($direction == 'stop') {
$url += 'ptzStopRun';
}
else {
$url += 'ptzStopRun';
}
$url += '&usr=' + $("#login").val() + '&pwd=' + $("#password").val();
// console.log($url);
$.ajax({
type: "GET",
cache: false,
crossDomain: true,
contentType: "text/xml",
async: false,
url: $url,
dataType: "jsonp",
success: function () {
},
error: function () {
}
});
}
</script>
<h1>JQuery Foscam Camera Control Interface</h1>
In Chrome, enable VLC plugin by going to chrome://flags/#enable-npapi and enabling it, then restarting Chrome.
<table>
<tr>
<td>
Camera IP address: (ex: 10.1.10.80)
</td>
<td>
<input type="text" width="50" id="ipAddress" value="digitalagora.noip.me">
</td>
</tr>
<tr>
<td>
Camera port: (ex: 88)
</td>
<td>
<input type="text" width="5" id="cameraPort" value="88">
</td>
</tr>
<tr>
<td>
Login user id:
</td>
<td>
<input type="text" width="30" id="login" value="pi">
</td>
</tr>
<tr>
<td>
Password:
</td>
<td>
<input type="text" width="30" id="password" value="raspberry">
</td>
</tr>
<tr>
<td>
Video feed name: (ex: videoMain)
</td>
<td>
<input type="text" width="30" id="videoFeedName" value="videoMain">
</td>
</tr>
<tr>
<td>
</td>
<td style="text-align: center">
<input type="button" id="startVideoButton" value="Start Video">
<input type="button" id="stopVideoButton" value="Stop Video">
</td>
</tr>
</table>
<br/>
<table class="outlined">
<tr>
<td colspan="3" style="text-align: center">
Instructions: Click a HOLD the button pressed to move the camera. Release button to stop camera motion.
</td>
</tr>
<tr>
<td colspan="3">
</td>
</tr>
<tr>
<td class="wide"></td>
<td class="wide"><input type="button" id="buttonUp" value="-== Move Up ==-"></td>
<td class="wide"></td>
</tr>
<tr>
<td class="wide"><input type="button" id="buttonLeft" value="-== Move Left ==-"></td>
<td width="640px" height="480px">
<EMBED TYPE="application/x-vlc-plugin" id="vlc" name="stream1" autoplay="yes" width="640" height="480"
loop="no" target="">
</EMBED>
</td>
<td class="wide"><input type="button" id="buttonRight" value="-== Move Right ==-"></td>
</tr>
<tr>
<td class="wide"></td>
<td class="wide"><input type="button" id="buttonDown" value="-== Move Down ==-"></td>
<td class="wide"></td>
</tr>
</table>
</body>
</html>