#!/bin/bash
# https://linuxconfig.org/how-to-create-a-new-virtual-machine-on-xenserver-using-command-line
NEW_VM_NAME="mytestbox"
DISKSIZE="10GiB"
# 1, 2, 3, 4, 5, 6, 7, 8
MAX_VIRTUAL_CPUS=1
# prompt user for specs:
read -ep "Enter the new box name, (ex: mytestbox) : " NEW_VM_NAME
read -ep "How many virtual CPUs, (ex: 1, 2, 4) : " -i "1" MAX_VIRTUAL_CPUS
read -ep "Hard drive size in gigs: (ex: 10) : " -i "10" GIGS_NUMBER
DISKSIZE="${GIGS_NUMBER}GiB"
read -ep "RAM size in gigs: (ex: 2) : " -i "2" RAM_NUMBER
RAMGIGS="${RAM_NUMBER}GiB"
echo "*********************************"
echo " box name: $NEW_VM_NAME "
echo " CPUs: $MAX_VIRTUAL_CPUS "
echo " DISK: $DISKSIZE"
echo " RAM: $RAMGIGS"
echo "*********************************"
# *****************************
# Don't change below
# *****************************
# Make sure "Ubuntu Bionic Beaver 18.04" exists in:
# xe template-list | grep name-label | grep "Ubuntu Bionic Beaver 18.04"
TEMPLATE="Ubuntu Bionic Beaver 18.04"
echo "1 Your template is: ${TEMPLATE}"
UUID=`xe vm-install template="${TEMPLATE}" new-name-label="${NEW_VM_NAME}"`
echo "2 Your template UUID is: ${UUID}"
# Use: xe cd-list to find out your .iso image name
ISO="ubuntu-18.04.4-server-amd64-unattended.iso"
echo "3 Your iso is: ${ISO}"
# use xe network-list
# and grab an eth interface uuid
NETWORKUUID=`xe network-list | sed -n '/eth0/{x;p;d;}; x' | head -n 1 | cut -d':' -f 2 | awk '{$1=$1;print}'`
echo "4 Your network uuid is: ${NETWORKUUID}"
# get the "Disk 0 VDI" uuid, which is the next line after VDI
VDI=`xe vm-disk-list --multiple vm="${NEW_VM_NAME}" | sed -n '/VDI/{n;p;}' | head -n 1 | cut -d':' -f 2 | awk '{$1=$1;print}'`
echo "5 Your VDI uuid is: ${VDI}"
xe vm-cd-add uuid=$UUID cd-name=$ISO device=1
xe vm-param-set HVM-boot-policy="BIOS order" uuid=$UUID
xe vif-create vm-uuid=$UUID network-uuid=$NETWORKUUID device=0
# Specify RAM amount to be used by this virtual machine.
#xe vm-memory-limits-set dynamic-max=2000MiB dynamic-min=512MiB static-max=2000MiB static-min=512MiB uuid=$UUID
xe vm-memory-limits-set dynamic-max=${RAMGIGS} dynamic-min=1GiB static-max=${RAMGIGS} static-min=1GiB uuid=$UUID
# set CPU parameters
xe vm-param-set VCPUs-max=$MAX_VIRTUAL_CPUS uuid=$UUID
xe vm-param-set VCPUs-at-startup=$MAX_VIRTUAL_CPUS uuid=$UUID
xe vm-param-set platform:cores-per-socket="1" uuid=$UUID
# update the size of your virtual disk
echo "updating size of your VM to $DISKSIZE"
xe vdi-resize uuid=$VDI disk-size=$DISKSIZE
# start the new VM
echo "starting your vm now..."
xe vm-start uuid=$UUID
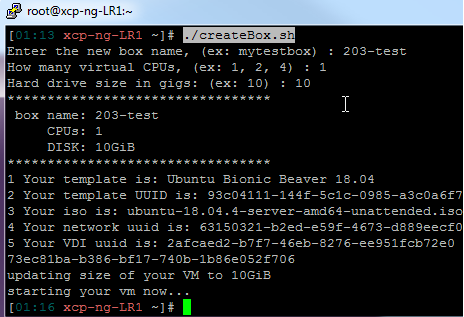
Watch it get created:
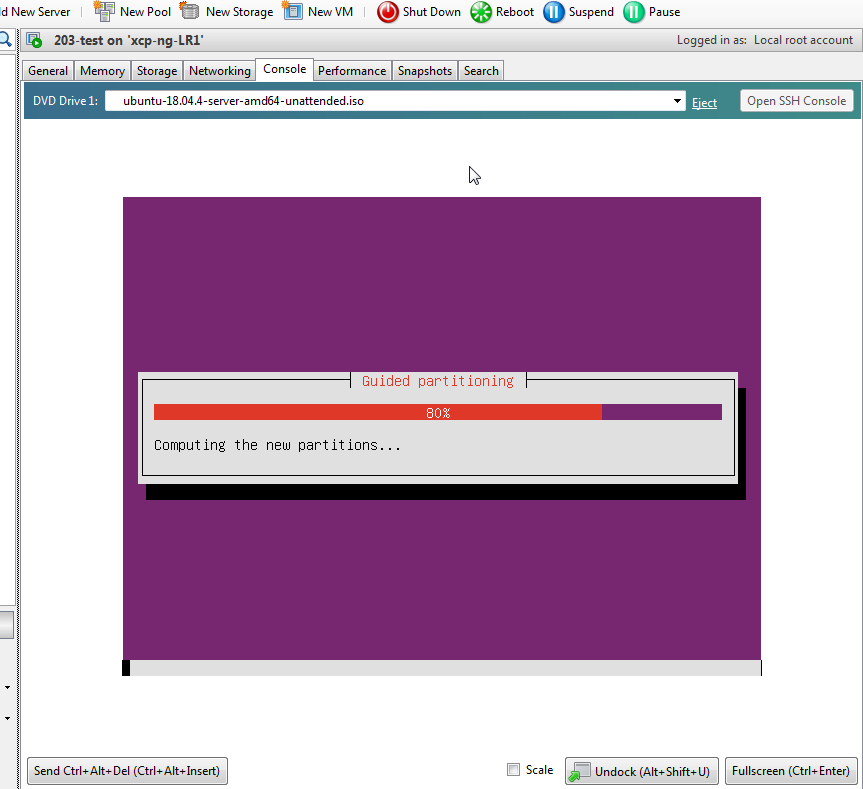