Scripting the creation of VMs will save you a ton of hours, and it’s quite awesome.
This works best if you have an Ubuntu UNATTENDED install ISO created, which installs the whole OS without any input.
Example script run, one-liner:
./createBox2021.sh k1 1 10 2 86:a9:a3:b0:99:01
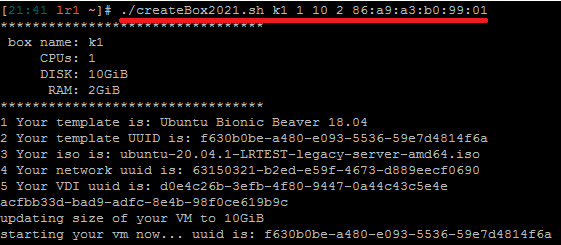
Or with menu selections:
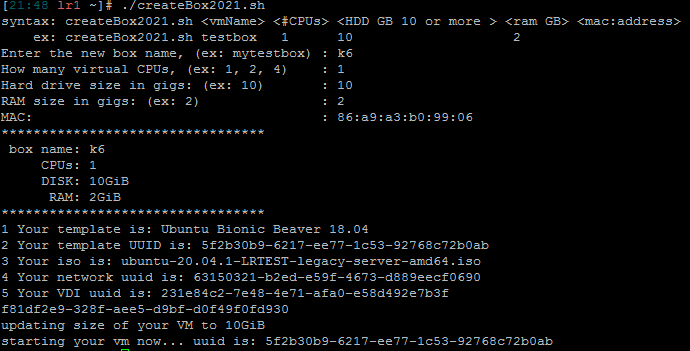
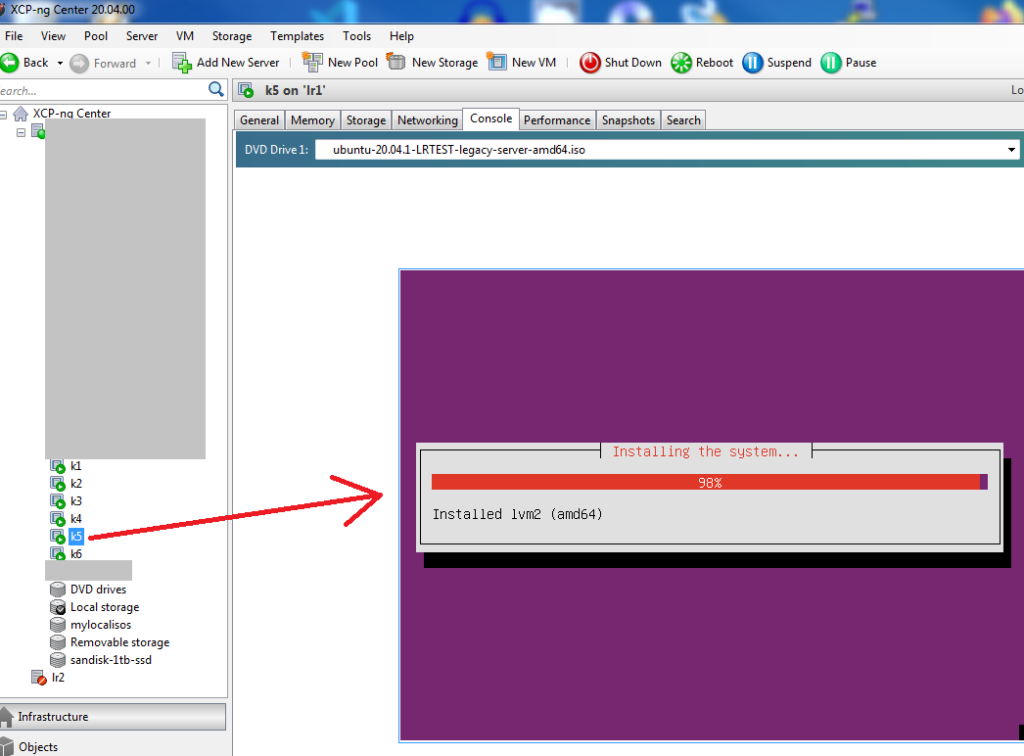
You’ll need your install ISO set up here. Use: xe cd-list to find out your .iso image name.
ISO="ubuntu-20.04.1-LRTEST-legacy-server-amd64.iso"
and you’ll need to pick your storage repository here, find the uuid by running “xe sr-list”:
SRUUID=885d75ed-08ea-ddd8-0044-8c66ef04000d
Once these are set up in the script, run it with or without arguments.
The script:
#!/bin/bash
# https://linuxconfig.org/how-to-create-a-new-virtual-machine-on-xenserver-using-command-line
# defaults
NEW_VM_NAME="mytestbox"
DISKSIZE="10GiB"
# 1, 2, 3, 4, 5, 6, 7, 8
MAX_VIRTUAL_CPUS=1
# if the number of arguments isn't right, then skip this section
if [ $# -ne 5 ]; then
echo "syntax: createBox2021.sh <vmName> <#CPUs> <HDD GB 10 or more > <ram GB> <mac:address>"
echo " ex: createBox2021.sh testbox 1 10 2"
# prompt user for specs:
read -ep "Enter the new box name, (ex: mytestbox) : " NEW_VM_NAME
read -ep "How many virtual CPUs, (ex: 1, 2, 4) : " -i "1" MAX_VIRTUAL_CPUS
read -ep "Hard drive size in gigs: (ex: 10) : " -i "10" GIGS_NUMBER
DISK_GIGS="${GIGS_NUMBER}GiB"
read -ep "RAM size in gigs: (ex: 2) : " -i "2" RAM_NUMBER
RAM_GIGS="${RAM_NUMBER}GiB"
read -ep "MAC: : " -i "86:a9:a3:b0:99:01" MYMAC
else
NEW_VM_NAME=$1
MAX_VIRTUAL_CPUS=$2
DISK_GIGS="${3}GiB"
RAM_GIGS="${4}GiB"
MYMAC="${5}"
fi
echo "*********************************"
echo " box name: $NEW_VM_NAME "
echo " CPUs: $MAX_VIRTUAL_CPUS "
echo " DISK: $DISK_GIGS"
echo " RAM: $RAM_GIGS"
echo "*********************************"
# *****************************
# Don't change below
# *****************************
# Make sure "Ubuntu Bionic Beaver 18.04" exists in:
# xe template-list | grep name-label | grep "Ubuntu Bionic Beaver 18.04"
TEMPLATE="Ubuntu Bionic Beaver 18.04"
echo "1 Your template is: ${TEMPLATE}"
# Which storage repository to install on. Find the uuid by running "xe sr-list"
SRUUID=885d75ed-08ea-ddd8-0044-8c66ef04000d
UUID=`xe vm-install template="${TEMPLATE}" new-name-label="${NEW_VM_NAME}" sr-uuid="${SRUUID}" `
echo "2 Your template UUID is: ${UUID}"
# Use: xe cd-list to find out your .iso image name
ISO="ubuntu-20.04.1-LRTEST-legacy-server-amd64.iso"
echo "3 Your iso is: ${ISO}"
# use xe network-list
# and grab an eth interface uuid
NETWORKUUID=`xe network-list | sed -n '/eth0/{x;p;d;}; x' | head -n 1 | cut -d':' -f 2 | awk '{$1=$1;print}'`
echo "4 Your network uuid is: ${NETWORKUUID}"
# get the "Disk 0 VDI" uuid, which is the next line after VDI
VDI=`xe vm-disk-list --multiple vm="${NEW_VM_NAME}" | sed -n '/VDI/{n;p;}' | head -n 1 | cut -d':' -f 2 | awk '{$1=$1;print}'`
echo "5 Your VDI uuid is: ${VDI}"
xe vm-cd-add uuid=$UUID cd-name=$ISO device=1
xe vm-param-set HVM-boot-policy="BIOS order" uuid=$UUID
xe vif-create vm-uuid=$UUID network-uuid=$NETWORKUUID mac=$MYMAC device=0
# Specify RAM amount to be used by this virtual machine.
xe vm-memory-limits-set dynamic-max=${RAM_GIGS} dynamic-min=${RAM_GIGS} static-max=${RAM_GIGS} static-min=${RAM_GIGS} uuid=$UUID
# set CPU parameters
xe vm-param-set VCPUs-max=$MAX_VIRTUAL_CPUS uuid=$UUID
xe vm-param-set VCPUs-at-startup=$MAX_VIRTUAL_CPUS uuid=$UUID
xe vm-param-set platform:cores-per-socket="1" uuid=$UUID
# update the size of your virtual disk
echo "updating size of your VM to $DISK_GIGS"